NvInputHandler_CameraFly Class Reference
Maps touch, mouse and gamepad into useful state for first person-ish fly camera (camera-relative pitch and translation, world yaw). More...
#include <NvInputHandler_CameraFly.h>
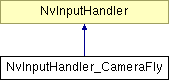
Public Member Functions | |
virtual void | reset () |
Reset. | |
virtual void | update (float deltaTime) |
Update the state based on the current inputs, velocities, and delta time. | |
virtual bool | processPointer (NvInputDeviceType::Enum device, NvPointerActionType::Enum action, uint32_t modifiers, int32_t count, NvPointerEvent *points) |
Pointer event input. | |
virtual bool | processGamepad (uint32_t changedPadFlags, NvGamepad &pad) |
Gamepad event input. | |
virtual bool | processKey (uint32_t code, NvKeyActionType::Enum action) |
Key event input. | |
void | setPosition (const nv::vec3f &pos) |
Sets the current position of the camera in world space. | |
const nv::vec3f & | getPosition () const |
Retrieves the current position of the camera in world space. | |
void | setPitch (float pitch) |
Sets the current pitch of the camera. | |
float | getPitch () const |
Retrieves the current pitch of the camera. | |
void | setYaw (float yaw) |
Sets the current yaw of the camera. | |
float | getYaw () const |
Retrieves the current yaw of the camera. | |
nv::vec3f | getLookVector () const |
Returns the current look-at vector of the camera. | |
const nv::matrix4f & | getCameraMatrix () const |
Retrieves the current world matrix of the camera's transform. | |
const nv::matrix4f & | getViewMatrix () const |
Retrieves the current view matrix from the camera. | |
void | setMouseRotationSpeed (float speed) |
Sets the speed of rotation that maps to mouse movements. | |
void | setMouseTranslationSpeed (float speed) |
Sets the speed of translation that maps to mouse movements. | |
void | setKeyboardRotationSpeed (float speed) |
Sets the speed of rotation that maps to key presses. | |
void | setKeyboardTranslationSpeed (float speed) |
Sets the speed of translation that maps to key presses. | |
void | setGamepadRotationSpeed (float speed) |
Sets the speed of rotation that maps to gamepad sticks. | |
void | setGamepadTranslationSpeed (float speed) |
Sets the speed of translation that maps to gamepad sticks. | |
Protected Member Functions | |
void | updateMatrices () |
Update the camera and view matrices. | |
Protected Attributes | |
nv::vec3f | m_currentPos |
float | m_currentPitch |
float | m_currentYaw |
nv::matrix4f | m_currentCameraMat |
nv::matrix4f | m_currentViewMat |
uint8_t | m_touchPointsCount |
nv::vec2f | m_lastInput |
float | m_lastMultitouchDist |
float | m_rotSpeed_Mouse |
float | m_transSpeed_Mouse |
float | m_xRotDelta_Mouse |
float | m_yRotDelta_Mouse |
float | m_zRotDelta_Mouse |
float | m_xTransDelta_Mouse |
float | m_yTransDelta_Mouse |
float | m_zTransDelta_Mouse |
float | m_rotSpeed_KB |
float | m_transSpeed_KB |
bool | m_accelerate_KB |
int8_t | m_xPlusRotVel_KB |
int8_t | m_yPlusRotVel_KB |
int8_t | m_zPlusRotVel_KB |
int8_t | m_xNegRotVel_KB |
int8_t | m_yNegRotVel_KB |
int8_t | m_zNegRotVel_KB |
int8_t | m_yPlusTransVel_KB |
int8_t | m_xPlusTransVel_KB |
int8_t | m_zPlusTransVel_KB |
int8_t | m_yNegTransVel_KB |
int8_t | m_xNegTransVel_KB |
int8_t | m_zNegTransVel_KB |
int32_t | m_lastActiveGamepad |
float | m_rotSpeed_GP |
float | m_transSpeed_GP |
bool | m_accelerate_GP |
float | m_xRotVel_GP |
float | m_yRotVel_GP |
float | m_zRotVel_GP |
float | m_xTransVel_GP |
float | m_yTransVel_GP |
float | m_zTransVel_GP |
Detailed Description
Maps touch, mouse and gamepad into useful state for first person-ish fly camera (camera-relative pitch and translation, world yaw).Member Function Documentation
const nv::matrix4f& NvInputHandler_CameraFly::getCameraMatrix | ( | ) | const [inline] |
Retrieves the current world matrix of the camera's transform.
- Returns:
- A matrix containing a representation of the camera's current position and orientation in world space
nv::vec3f NvInputHandler_CameraFly::getLookVector | ( | ) | const [inline] |
Returns the current look-at vector of the camera.
- Returns:
- the look-at vector
float NvInputHandler_CameraFly::getPitch | ( | ) | const [inline] |
Retrieves the current pitch of the camera.
- Returns:
- The current pitch of the camera, in radians
const nv::vec3f& NvInputHandler_CameraFly::getPosition | ( | ) | const [inline] |
Retrieves the current position of the camera in world space.
- Returns:
- The current world-space position of the camera
const nv::matrix4f& NvInputHandler_CameraFly::getViewMatrix | ( | ) | const [inline] |
Retrieves the current view matrix from the camera.
- Returns:
- A matrix containing a representation of the transform required to transform a position from world space into camera view space
float NvInputHandler_CameraFly::getYaw | ( | ) | const [inline] |
Retrieves the current yaw of the camera.
- Returns:
- The current yaw of the camera, in radians
virtual bool NvInputHandler_CameraFly::processGamepad | ( | uint32_t | changedPadFlags, | |
NvGamepad & | pad | |||
) | [virtual] |
Gamepad event input.
Used to pass gamepad state to the handler. The signature explicitly matches that of the input callbacks used to provide input to an app or app framework for ease of calling
- Parameters:
-
[in] changedPadFlags Flags indicating which gamepads have changed state [in] pad The gamepad device
- Returns:
- True if the event was used and "eaten" by the input transformer and should not be processed by any other input system.
Implements NvInputHandler.
virtual bool NvInputHandler_CameraFly::processKey | ( | uint32_t | code, | |
NvKeyActionType::Enum | action | |||
) | [virtual] |
Key event input.
Used to pass key input events to the handler. The signature explicitly matches that of the input callbacks used to provide input to an app or app framework for ease of calling
- Parameters:
-
[in] code The input keycode [in] action The input action
- Returns:
- True if the event was used and "eaten" by the input transformer and should not be processed by any other input system.
Implements NvInputHandler.
virtual bool NvInputHandler_CameraFly::processPointer | ( | NvInputDeviceType::Enum | device, | |
NvPointerActionType::Enum | action, | |||
uint32_t | modifiers, | |||
int32_t | count, | |||
NvPointerEvent * | points | |||
) | [virtual] |
Pointer event input.
Used to pass pointer input events to the handler. The signature explicitly matches that of the input callbacks used to provide input to an app or app framework for ease of calling
- Parameters:
-
[in] device The input device [in] action The input action [in] modifiers The input modifiers [in] count The number of elements in the points array [in] points The input event points
- Returns:
- True if the event was used and "eaten" by the input handler and should not be processed by any other input system.
Implements NvInputHandler.
virtual void NvInputHandler_CameraFly::reset | ( | ) | [virtual] |
Reset.
Reset all input values to default/starting points, restoring initial view.
Implements NvInputHandler.
void NvInputHandler_CameraFly::setGamepadRotationSpeed | ( | float | speed | ) | [inline] |
Sets the speed of rotation that maps to gamepad sticks.
- Parameters:
-
speed Speed of gamepad driven rotation, in radians per second at full deflection
void NvInputHandler_CameraFly::setGamepadTranslationSpeed | ( | float | speed | ) | [inline] |
Sets the speed of translation that maps to gamepad sticks.
- Parameters:
-
speed Speed of gamepad driven translation, in meters per second at full deflection
void NvInputHandler_CameraFly::setKeyboardRotationSpeed | ( | float | speed | ) | [inline] |
Sets the speed of rotation that maps to key presses.
- Parameters:
-
speed Speed of keyboard driven rotation, in radians per second
void NvInputHandler_CameraFly::setKeyboardTranslationSpeed | ( | float | speed | ) | [inline] |
Sets the speed of translation that maps to key presses.
- Parameters:
-
speed Speed of keyboard driven translation, in meters per second
void NvInputHandler_CameraFly::setMouseRotationSpeed | ( | float | speed | ) | [inline] |
Sets the speed of rotation that maps to mouse movements.
- Parameters:
-
speed Speed of mouse driven rotation, in radians per pixel
void NvInputHandler_CameraFly::setMouseTranslationSpeed | ( | float | speed | ) | [inline] |
Sets the speed of translation that maps to mouse movements.
- Parameters:
-
speed Speed of mouse driven translation, in meters per pixel
void NvInputHandler_CameraFly::setPitch | ( | float | pitch | ) | [inline] |
Sets the current pitch of the camera.
- Parameters:
-
pitch New pitch of the camera, in radians
void NvInputHandler_CameraFly::setPosition | ( | const nv::vec3f & | pos | ) | [inline] |
Sets the current position of the camera in world space.
- Parameters:
-
[in] pos The world position at which to place the camera
void NvInputHandler_CameraFly::setYaw | ( | float | yaw | ) | [inline] |
Sets the current yaw of the camera.
- Parameters:
-
pitch New yaw of the camera, in radians
virtual void NvInputHandler_CameraFly::update | ( | float | deltaTime | ) | [virtual] |
Update the state based on the current inputs, velocities, and delta time.
- Parameters:
-
[in] deltaTime the time since the last call to update, in seconds
Implements NvInputHandler.
The documentation for this class was generated from the following file: