NvVkContext Class Reference
A wrapper for most major Vulkan objects used by an application. More...
#include <NvVkContext.h>
Inheritance diagram for NvVkContext:
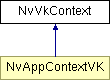
Classes | |
struct | DebugMarkerScope |
struct | FrameInfo |
Public Types | |
enum | CmdBufferIndex { CMD_ONSCREEN = 0, CMD_UI_FRAMEWORK, CMD_COUNT } |
Public Member Functions | |
NvVkContext (const NvVKConfiguration &config) | |
VkInstance | instance () |
VkInstance access. | |
VkDevice | device () const |
Device access. | |
VkQueue | queue () |
Queue access. | |
uint32_t | queueFamilyIndex () |
Queue family access. | |
uint32_t | queueIndex () |
Queue index access. | |
VkPhysicalDevice & | physicalDevice () |
VkPhysicalDevice access. | |
VkPhysicalDeviceLimits & | physicalDeviceLimits () |
VkPhysicalDeviceLimits access. | |
VkPhysicalDeviceProperties & | physicalDeviceProperties () |
VkPhysicalDeviceProperties access. | |
VkPhysicalDeviceFeatures & | physicalDeviceFeatures () |
VkPhysicalDeviceFeatures access. | |
VkPhysicalDeviceMemoryProperties & | physicalDeviceMemoryProperties () |
VkPhysicalDeviceMemoryProperties access. | |
virtual NvVkRenderTarget * | mainRenderTarget ()=0 |
Get the on-screen (or main) render target. | |
VkResult | allocMemAndBindImage (NvVkImage &image, VkFlags memProps=0) |
VkResult | createImage (VkImageCreateInfo &info, NvVkImage &image, VkFlags memProps=0) |
VkResult | allocMemAndBindBuffer (NvVkBuffer &buffer, VkFlags memProps=0) |
VkResult | createAndFillBuffer (size_t size, VkFlags usage, VkFlags memProps, NvVkBuffer &buffer, const void *data=NULL, NvVkStagingBuffer *staging=NULL) |
bool | uploadTextureFromDDSFile (const char *filename, NvVkTexture &tex) |
Creates a texture from an asset-based DDS file. | |
bool | uploadTextureFromFile (const char *filename, NvVkTexture &tex) |
Creates a texture from an asset-based image file. | |
bool | uploadTextureFromDDSData (const char *ddsData, int32_t length, NvVkTexture &tex) |
Creates a texture from preloaded data in DDS file format. | |
bool | uploadTexture (const NvImage *image, NvVkTexture &tex) |
Creates a texture from an in-memory NvImage. | |
uint32_t | createShadersFromSourceString (const std::string &sourceText, VkPipelineShaderStageCreateInfo *shaders, uint32_t maxShaders) |
Deprecated: Only supported on platforms/drivers that can accept GLSL directly. | |
uint32_t | createShadersFromBinaryBlob (uint32_t *data, uint32_t leng, VkPipelineShaderStageCreateInfo *shaders, uint32_t maxShaders) |
Load SPIR-V shaders from binary file data (generated from the glsl2spirv tool). | |
VkResult | transitionImageLayout (VkImage &image, VkImageAspectFlags aspect, VkImageLayout oldLayout, VkImageLayout newLayout, VkAccessFlagBits inSrcAccessmask=VK_ACCESS_COLOR_ATTACHMENT_READ_BIT, VkAccessFlagBits inDstAccessmask=VK_ACCESS_COLOR_ATTACHMENT_WRITE_BIT) |
VkCommandBuffer | getMainCommandBuffer (CmdBufferIndex index=CMD_ONSCREEN) |
bool | submitMainCommandBuffer (CmdBufferIndex index=CMD_ONSCREEN) |
VkCommandBuffer | createCmdBuffer (VkCommandPool pool, bool primary) |
void | purgeTempCmdBuffers () |
VkCommandBuffer | beginTempCmdBuffer () |
VkResult | doneWithTempCmdBufferSubmit (VkCommandBuffer &cmd, VkFence *fence=NULL) |
void | doneWithTempCmdBuffer (VkCommandBuffer &cmd) |
void | destroyPastFrameCmdBuffers () |
NvVkStagingBuffer & | stagingBuffer () |
NvGPUTimerVK & | getFrameTimer () |
Protected Types | |
enum | { MAX_BUFFERED_FRAMES = 2 } |
Protected Member Functions | |
VkResult | fillBuffer (NvVkStagingBuffer *staging, NvVkBuffer &buffer, size_t offset, size_t size, const void *data) |
VkShaderModule | createShader (const char *shaderSource, VkShaderStageFlagBits inStage) |
virtual bool | reshape (int32_t &w, int32_t &h) |
virtual VkSemaphore * | getWaitSync ()=0 |
virtual VkSemaphore * | getSignalSync ()=0 |
Protected Attributes | |
VkInstance | _instance |
VkPhysicalDevice | _physicalDevice |
VkPhysicalDeviceProperties | _physicalDeviceProperties |
VkPhysicalDeviceMemoryProperties | _physicalDeviceMemoryProperties |
VkPhysicalDeviceLimits | _physicalDeviceLimits |
VkPhysicalDeviceFeatures | _physicalDeviceFeatures |
VkPhysicalDeviceFeatures | _physicalDeviceFeaturesEnabled |
VkDevice | _device |
VkQueue | _queue |
VkQueueFamilyProperties | _queueProperties |
uint32_t | _queueFamilyIndex |
uint32_t | _queueIndex |
VkCommandPool | mTempCmdPool |
FrameInfo | mFrames [MAX_BUFFERED_FRAMES] |
uint32_t | mCurrFrameIndex |
NvVkStagingBuffer | mStaging |
NvGPUTimerVK * | m_frameTimer |
std::vector < VkExtensionProperties > | mInstanceExtensionsProperties |
std::vector< VkLayerProperties > | mInstanceLayerProperties |
std::vector < VkExtensionProperties > | mPhysicalDeviceExtensionsProperties |
std::vector< VkLayerProperties > | mPhysicalDeviceLayerProperties |
std::set< std::string > | mCombinedExtensionNames |
std::set< std::string > | mCombinedLayerNames |
NvVKConfiguration | mConfiguration |
bool | mSupportsDebugMarkers |
Detailed Description
A wrapper for most major Vulkan objects used by an application.Member Function Documentation
uint32_t NvVkContext::createShadersFromBinaryBlob | ( | uint32_t * | data, | |
uint32_t | leng, | |||
VkPipelineShaderStageCreateInfo * | shaders, | |||
uint32_t | maxShaders | |||
) |
Load SPIR-V shaders from binary file data (generated from the glsl2spirv tool).
- Parameters:
-
[in] data the binary data with multiple shaders [in] leng the size of the data in bytes in/out] shaders pointer to an array of shader stage create info structs into which the shaders will be written. Array must be at least as large as the number of shader stages in the file [in] maxShaders the number of shader structs in the array
- Returns:
- the number of shader stages loaded, or zero on failure.
bool NvVkContext::uploadTexture | ( | const NvImage * | image, | |
NvVkTexture & | tex | |||
) |
Creates a texture from an in-memory NvImage.
- Parameters:
-
[in] image the image to load [out] tex the loaded texture
- Returns:
- true on success and false on failure
bool NvVkContext::uploadTextureFromDDSData | ( | const char * | ddsData, | |
int32_t | length, | |||
NvVkTexture & | tex | |||
) |
Creates a texture from preloaded data in DDS file format.
- Parameters:
-
[in] ddsData pointer to the DDS file data to load [out] tex the loaded texture
- Returns:
- true on success and false on failure
bool NvVkContext::uploadTextureFromDDSFile | ( | const char * | filename, | |
NvVkTexture & | tex | |||
) |
Creates a texture from an asset-based DDS file.
- Parameters:
-
[in] filename the asset-path of the DDS file to load [out] tex the loaded texture
- Returns:
- true on success and false on failure
bool NvVkContext::uploadTextureFromFile | ( | const char * | filename, | |
NvVkTexture & | tex | |||
) |
Creates a texture from an asset-based image file.
- Parameters:
-
[in] filename the asset-path of the image file to load [out] tex the loaded texture
- Returns:
- true on success and false on failure
The documentation for this class was generated from the following file: