NvUIText Class Reference
A UI element that renders text strings to the view. More...
#include <NvUI.h>
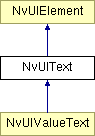
Public Member Functions | |
NvUIText (const char *str, NvUIFontFamily::Enum font, float size, NvUITextAlign::Enum halign) | |
Default constructor for onscreen text element. | |
virtual void | SetAlignment (NvUITextAlign::Enum halign) |
Set the horizontal alignment of the text. | |
virtual void | SetAlpha (float alpha) |
Set the alpha transparency of the text. | |
virtual void | SetColor (const NvPackedColor &color) |
Set the overall color of the text. | |
virtual void | SetShadow (char offset=DEFAULT_SHADOW_OFFSET, NvPackedColor color=NV_PC_PREDEF_BLACK) |
Enable a drop-shadow visual under the text string. | |
virtual void | SetTextBox (float width, float height, uint32_t lines, uint32_t dots) |
Assign a box the text will render within. | |
virtual void | SetDimensions (float w, float h) |
Override so if a box has been set, we update the width and height with these values. | |
virtual void | Draw (const NvUIDrawState &drawState) |
Make proper calls to the text rendering system to draw our text to the viewport. | |
void | SetString (const char *in) |
Set the string to be drawn. | |
virtual void | SetFontSize (float size) |
Set the font size to use for our text. | |
float | GetFontSize () |
Get our font size value. | |
float | GetStringPixelWidth () |
Get the approximate output pixel width calculated for our text. | |
void | SetDrawnChars (int32_t count) |
Set an explicit character count truncation for drawing our text. | |
Static Public Member Functions | |
static uint8_t | GetFontID (NvUIFontFamily::Enum font) |
Static helper method to get the specific font ID for a given font family enum. | |
static bool | StaticInit (float width=1280, float height=720) |
Static helper method for initializing underlying text system with current view size. | |
static void | StaticCleanup () |
Static helper method for cleaning up any static held items in the text system. | |
Protected Attributes | |
NvBFText * | m_bftext |
The NvBitFont BFText object that does actual text rendering. | |
float | m_size |
Local cache of the original font size. | |
NvPackedColor | m_color |
Modulation of the text with an RGB color. | |
bool | m_wrap |
Whether we wrap or truncate if exceed drawable width. | |
Static Protected Attributes | |
static const char | DEFAULT_SHADOW_OFFSET = 3 |
The default/canonical shadow offset value. |
Detailed Description
A UI element that renders text strings to the view.
This class wrappers rendering of a string to the screen using NvBitFont
.
It exposes all the matching functionality, including font, size, color, drop-shadow, alignment 'text box', and multi-line output support.
- See also:
- NvBitFont.h
Constructor & Destructor Documentation
NvUIText::NvUIText | ( | const char * | str, | |
NvUIFontFamily::Enum | font, | |||
float | size, | |||
NvUITextAlign::Enum | halign | |||
) |
Default constructor for onscreen text element.
- Parameters:
-
str Text string to display. font Font family to use for this text. size Font size to use for this text. halign Alignment to use for this text.
Member Function Documentation
virtual void NvUIText::Draw | ( | const NvUIDrawState & | drawState | ) | [virtual] |
Make proper calls to the text rendering system to draw our text to the viewport.
Reimplemented from NvUIElement.
Reimplemented in NvUIValueText.
static uint8_t NvUIText::GetFontID | ( | NvUIFontFamily::Enum | font | ) | [static] |
Static helper method to get the specific font ID for a given font family enum.
float NvUIText::GetFontSize | ( | ) | [inline] |
Get our font size value.
float NvUIText::GetStringPixelWidth | ( | ) |
Get the approximate output pixel width calculated for our text.
virtual void NvUIText::SetAlignment | ( | NvUITextAlign::Enum | halign | ) | [virtual] |
Set the horizontal alignment of the text.
virtual void NvUIText::SetAlpha | ( | float | alpha | ) | [virtual] |
Set the alpha transparency of the text.
Reimplemented from NvUIElement.
Reimplemented in NvUIValueText.
virtual void NvUIText::SetColor | ( | const NvPackedColor & | color | ) | [virtual] |
virtual void NvUIText::SetDimensions | ( | float | w, | |
float | h | |||
) | [virtual] |
Override so if a box has been set, we update the width and height with these values.
Reimplemented from NvUIElement.
Reimplemented in NvUIValueText.
void NvUIText::SetDrawnChars | ( | int32_t | count | ) |
Set an explicit character count truncation for drawing our text.
This is handy helper function for doing simple 'type on' animated visuals.
virtual void NvUIText::SetFontSize | ( | float | size | ) | [virtual] |
virtual void NvUIText::SetShadow | ( | char | offset = DEFAULT_SHADOW_OFFSET , |
|
NvPackedColor | color = NV_PC_PREDEF_BLACK | |||
) | [virtual] |
Enable a drop-shadow visual under the text string.
- Parameters:
-
offset A positive (down+right) or negative (up+left) pixel offset for shadow. Default is +2 pixels. Set 0 to disable. color An NvPackedColor for what color the drop-shadow should be. Default is black.
Reimplemented in NvUIValueText.
void NvUIText::SetString | ( | const char * | in | ) |
Set the string to be drawn.
virtual void NvUIText::SetTextBox | ( | float | width, | |
float | height, | |||
uint32_t | lines, | |||
uint32_t | dots | |||
) | [virtual] |
Assign a box the text will render within.
The box will be used to align, wrap, and crop the text.
-
If
lines
is 1, only a single line will be drawn, cropped at the box width. -
If
lines
is 0, we will wrap and never crop. -
If
lines
is >1, we will wrap for that many lines, and crop on the final line. -
If
dots
is a valid character, when we crop we won't simply truncate, will will back up enough to fit in three of thedots
character as ellipses. - When a box is active, all alignment settings on the text become relative to the box rather than the view.
- If width or height is zero, the box will be cleared/disabled.
- Parameters:
-
width Set the width of the box height Set the height of the box lines Set the number of vertical lines allowed for the text dots Set the character to use as ellipses
Reimplemented in NvUIValueText.
static void NvUIText::StaticCleanup | ( | ) | [static] |
Static helper method for cleaning up any static held items in the text system.
static bool NvUIText::StaticInit | ( | float | width = 1280 , |
|
float | height = 720 | |||
) | [static] |
Static helper method for initializing underlying text system with current view size.
Member Data Documentation
const char NvUIText::DEFAULT_SHADOW_OFFSET = 3 [static, protected] |
The default/canonical shadow offset value.
NvBFText* NvUIText::m_bftext [protected] |
The NvBitFont BFText object that does actual text rendering.
float NvUIText::m_size [protected] |
Local cache of the original font size.
bool NvUIText::m_wrap [protected] |
Whether we wrap or truncate if exceed drawable width.
The documentation for this class was generated from the following file: