NvUIValueText Class Reference
A UI element that converts numeric values to text and draws title and value. More...
#include <NvUI.h>
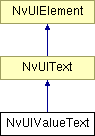
Public Member Functions | |
NvUIValueText (const char *str, NvUIFontFamily::Enum font, float size, NvUITextAlign::Enum titleAlign, float value, uint32_t precision, NvUITextAlign::Enum valueAlign, uint32_t actionCode=0) | |
Constructor for onscreen text element displaying a floating-point value. | |
NvUIValueText (const char *str, NvUIFontFamily::Enum font, float size, NvUITextAlign::Enum titleAlign, uint32_t value, NvUITextAlign::Enum valueAlign, uint32_t actionCode=0) | |
Constructor for onscreen text element displaying an integer value. | |
void | SetValueAlignment (NvUITextAlign::Enum halign) |
Set the horizontal alignment of the value text. | |
virtual void | SetAlpha (float alpha) |
Set the alpha transparency of both text strings. | |
virtual void | SetColor (const NvPackedColor &color) |
Set the overall color of both text strings. | |
void | SetValueColor (const NvPackedColor &color) |
Set the color of just the value text string. | |
virtual void | SetShadow (char offset=DEFAULT_SHADOW_OFFSET, NvPackedColor color=NV_PC_PREDEF_BLACK) |
Set drop-shadow features on the text strings. | |
virtual void | SetTextBox (float width, float height, uint32_t lines, uint32_t dots) |
Set a box the two strings will render within. | |
virtual void | SetDimensions (float w, float h) |
Override to set dimensions of both text strings. | |
virtual void | SetOrigin (float x, float y) |
Override to set origin of both text strings. | |
virtual void | Draw (const NvUIDrawState &drawState) |
Override to draw both title and value strings to the viewport. | |
virtual void | SetFontSize (float size) |
Set the font size to use for both text strings. | |
void | SetValue (float value) |
Set the value to be drawn as a string. | |
void | SetValue (uint32_t value) |
Set an integer value to be drawn as a string. | |
virtual NvUIEventResponse | HandleReaction (const NvUIReaction &react) |
Override to set our value if an appropriate reaction comes through. | |
Protected Attributes | |
float | m_value |
The current value. | |
bool | m_integral |
Whether or not the value is actually an integer. | |
uint32_t | m_precision |
Number of digits after decimal point to display, zero for default string formatting. | |
NvUIText * | m_valueText |
The NvUIText defining the output value text. | |
NvUITextAlign::Enum | m_titleAlign |
The alignment of the title output, so we can position value relative to title. | |
NvUITextAlign::Enum | m_valueAlign |
The alignment of the value output, so we can position relative to title. | |
uint32_t | m_action |
If nonzero, the action code we respond to for changing our value. |
Detailed Description
A UI element that converts numeric values to text and draws title and value.This class wrappers rendering of a title string and a value string to the screen leveraging NvUIText.
Valid combinations of alignments of the two items and the resulting output are:
- title: left, value: left ==> [TITLE VALUE ]
- title: left, value: right ==> [TITLE VALUE]
- title: right, value: right ==> [ VALUE TITLE]
- See also:
- NvUIText
Constructor & Destructor Documentation
NvUIValueText::NvUIValueText | ( | const char * | str, | |
NvUIFontFamily::Enum | font, | |||
float | size, | |||
NvUITextAlign::Enum | titleAlign, | |||
float | value, | |||
uint32_t | precision, | |||
NvUITextAlign::Enum | valueAlign, | |||
uint32_t | actionCode = 0 | |||
) |
Constructor for onscreen text element displaying a floating-point value.
- Parameters:
-
str Title string to display. font Font family to use for this text. size Font size to use for this text. titleAlign Alignment to use for the title text. value Starting value to show. precision Number of digits after decimal point to display. valueAlign Alignment to use for the value text. actionCode [optional] Reaction code to match to set our value.
NvUIValueText::NvUIValueText | ( | const char * | str, | |
NvUIFontFamily::Enum | font, | |||
float | size, | |||
NvUITextAlign::Enum | titleAlign, | |||
uint32_t | value, | |||
NvUITextAlign::Enum | valueAlign, | |||
uint32_t | actionCode = 0 | |||
) |
Constructor for onscreen text element displaying an integer value.
- Parameters:
-
str Title string to display. font Font family to use for this text. size Font size to use for this text. titleAlign Alignment to use for the title text. value Starting value to show. valueAlign Alignment to use for the value text. actionCode [optional] Reaction code to match to set our value.
Member Function Documentation
virtual void NvUIValueText::Draw | ( | const NvUIDrawState & | drawState | ) | [virtual] |
virtual NvUIEventResponse NvUIValueText::HandleReaction | ( | const NvUIReaction & | react | ) | [virtual] |
virtual void NvUIValueText::SetAlpha | ( | float | alpha | ) | [virtual] |
virtual void NvUIValueText::SetColor | ( | const NvPackedColor & | color | ) | [virtual] |
virtual void NvUIValueText::SetDimensions | ( | float | w, | |
float | h | |||
) | [virtual] |
virtual void NvUIValueText::SetFontSize | ( | float | size | ) | [virtual] |
virtual void NvUIValueText::SetOrigin | ( | float | x, | |
float | y | |||
) | [virtual] |
virtual void NvUIValueText::SetShadow | ( | char | offset = DEFAULT_SHADOW_OFFSET , |
|
NvPackedColor | color = NV_PC_PREDEF_BLACK | |||
) | [virtual] |
virtual void NvUIValueText::SetTextBox | ( | float | width, | |
float | height, | |||
uint32_t | lines, | |||
uint32_t | dots | |||
) | [virtual] |
void NvUIValueText::SetValue | ( | uint32_t | value | ) |
Set an integer value to be drawn as a string.
void NvUIValueText::SetValue | ( | float | value | ) |
Set the value to be drawn as a string.
void NvUIValueText::SetValueAlignment | ( | NvUITextAlign::Enum | halign | ) |
Set the horizontal alignment of the value text.
void NvUIValueText::SetValueColor | ( | const NvPackedColor & | color | ) |
Set the color of just the value text string.
Member Data Documentation
uint32_t NvUIValueText::m_action [protected] |
If nonzero, the action code we respond to for changing our value.
bool NvUIValueText::m_integral [protected] |
Whether or not the value is actually an integer.
uint32_t NvUIValueText::m_precision [protected] |
Number of digits after decimal point to display, zero for default string formatting.
NvUITextAlign::Enum NvUIValueText::m_titleAlign [protected] |
The alignment of the title output, so we can position value relative to title.
float NvUIValueText::m_value [protected] |
The current value.
NvUITextAlign::Enum NvUIValueText::m_valueAlign [protected] |
The alignment of the value output, so we can position relative to title.
NvUIText* NvUIValueText::m_valueText [protected] |
The NvUIText defining the output value text.
The documentation for this class was generated from the following file: